Block Side Menu
The Block Side Menu appears whenever you hover over a block, and is used to drag & drop the block as well as add new ones below it.

You can also click the drag handle (⠿
) in the Block Side Menu to open the Drag Handle Menu.
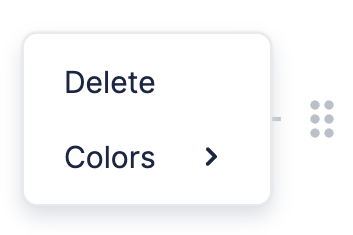
Custom Side Menu
If you want to change the items in the Side Menu, or replace it altogether, you can do that using a React component.
You can see how this is done in the example below, which has a custom Side Menu with two items. The first one deletes the selected block, while the second one is a drag handle, which opens a menu on click.
CustomSideMenu
is the component we use to replace the default Side Menu. You can see it's made up of a bunch of other components that are exported by BlockNote. Read on to Components to find out more about these.
After creating CustomSideMenu
, we tell BlockNote to use it inside BlockNoteView
. Changing UI Elements has more information about how this is done.
Custom Drag Handle Menu
If you want to change the items in the Drag Handle Menu, or replace it altogether, you can do that using a React component.
You can see how this is done in the example below, which has a custom Drag Handle Menu with two items. The first one deletes the selected block, while the second opens an alert.
CustomDragHandleMenu
is the component we use to replace the default Drag Handle Menu. You can see it's made up of a bunch of other components that are exported by BlockNote. Read on to Components to find out more about these.
Here, we're passing it as a prop to the DefaultSideMenu
component, but you can also pass it to the DragHandle
component we saw in the previous demo, if you don't want to use the default Side Menu layout.
After creating CustomDragHandleMenu
, we tell BlockNote to use it inside BlockNoteView
. Changing UI Elements has more information about how this is done.
Components
BlockNote provides React components that you can reuse, to easily create your menus while matching the default styling.
Default Components
BlockNote exports all components used to create the default layout - both the menu itself and the items in it. Head to the default Side Menu's source code and default Drag Handle Menu's source code to see all the components that you can use.
Custom Components
BlockNote also provides components that you can use to make your own menu items, which also match the default styling:
type SideMenuButtonProps = {
children: JSX.Element;
}
export const SideMenuButton = (props: SideMenuButtonProps) => ...;
// Contains all props that a regular button element would take, as well as all props from the Mantine `Menu.Item` component.
type DragHandleMenuItemProps = PolymorphicComponentProps<"button"> & MenuItemProps
export const DragHandleMenuItem = (props: DragHandleMenuItemProps) => ...;